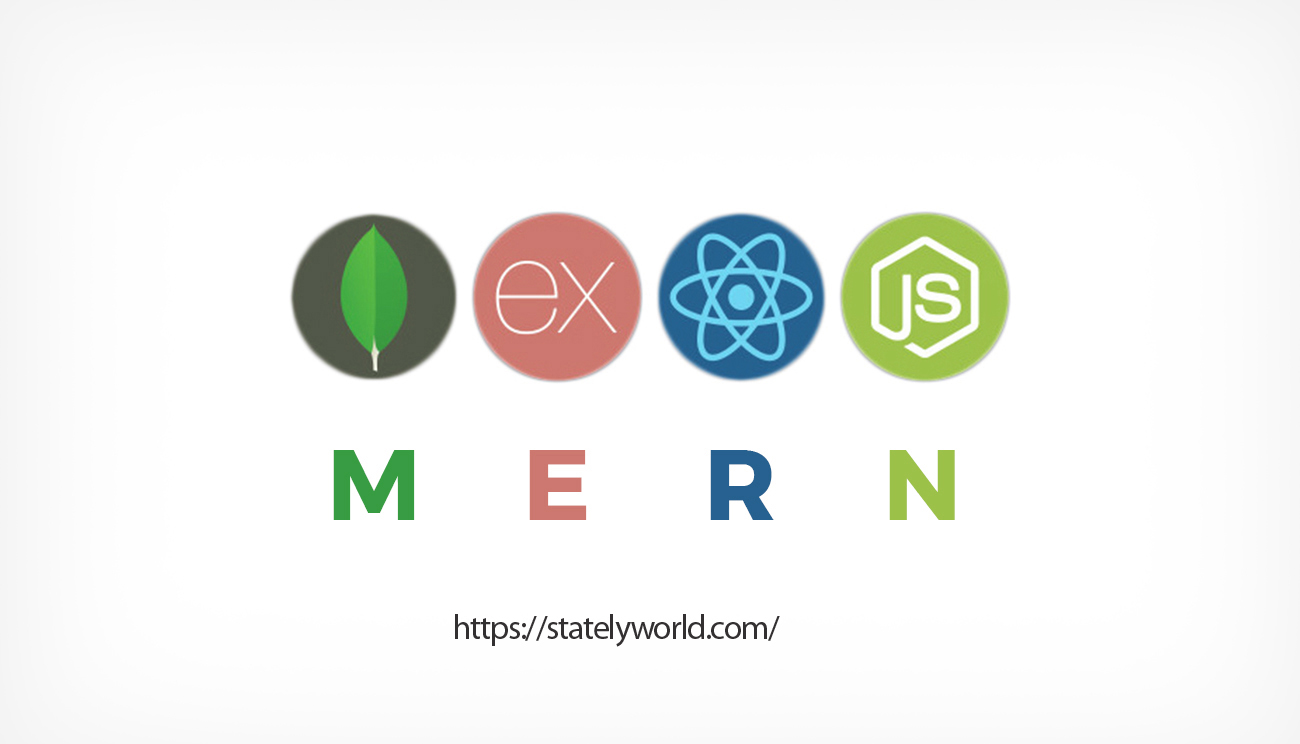
Express servers receive data from the client-side through the req
object in three instances: the req.params
, req.query
, and req.body
objects.
The req.params
object captures data based on the parameter specified in the URL. In your index.js
file, implement a GET
request with a parameter of '/:userid'
:
// GET https://example.com/user/1 app.get('/:userid', (req, res) => { console.log(req.params.userid) // "1" })
The req.params
object tells Express to output the result of a user’s id through the parameter '/:userid'
. Here, the GET
request to https://example.com/user/1
with the designated parameter logs into the console an id of "1"
.
To access a URL query string, apply the req.query
object to search, filter, and sort through data. In your index.js
file, include a GET
request to the route '/search'
:
// GET https://example.com/search?keyword=great-white app.get('/search', (req, res) => { console.log(req.query.keyword) // "great-white" })
Utilizing the req.query
object matches data loaded from the client side in a query conditional. In this case, the GET
request to the '/search'
route informs Express to match keywords in the search query to https://example.com
. The result from appending the .keyword
property to the req.query
object logs into the console, "great-white"
.
The req.body
object allows you to access data in a string or JSON object from the client side. You generally use the req.body
object to receive data through POST
and PUT
requests in the Express server.
In your index.js
file, set a POST
request to the route '/login'
:
// POST https://example.com/login // // { // "email": "user@example.com", // "password": "helloworld" // } app.post('/login', (req, res) => { console.log(req.body.email) // "user@example.com" console.log(req.body.password) // "helloworld" })
When a user inputs their email and password on the client side, the req.body
object stores that information and sends it to your Express server. Logging the req.body
object into the console results in the user’s email and password.
Now that you’ve examined ways to implement the req
object, let’s look at other approaches to integrate into your Express server.
Hi there I am so excited I found your webpage, I really found you by mistake, while I was looking on Aol
for something else, Regardless I am here now and would just like to say thank you for a fantastic post
and a all round interesting blog (I also love the theme/design),
I don’t have time to look over it all at the moment but I have saved it and also added
in your RSS feeds, so when I have time I will be back to read
a lot more, Please do keep up the great job.
I have learn several just right stuff here. Definitely worth
bookmarking for revisiting. I wonder how a lot effort you put to create any such fantastic informative site.